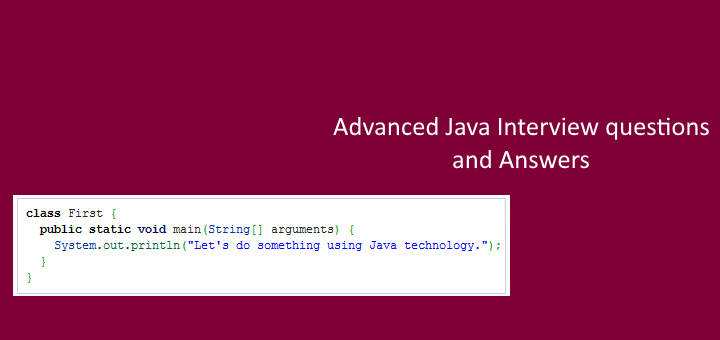
Java Interview Questions
Please go through important Java Interview Questions, solve them if you can, share it with your friends and save as bookmark for future reference!
Java Interview Questions:
What is difference between JDK,JRE and JVM?
JVM
JVM is an acronym for Java Virtual Machine, it is an abstract machine which provides the run-time environment in which java bytecode can be executed. It is a specification.
JVMs are available for many hardware and software platforms (so JVM is platform dependent).
JRE
JRE stands for Java Runtime Environment. It is the implementation of JVM.
JDK
JDK is an acronym for Java Development Kit. It physically exists. It contains JRE + development tools.
What is JIT compiler?
Just-In-Time(JIT) compiler: It is used to improve the performance. JIT compiles parts of the byte code that have similar functionality at the same time, and hence reduces the amount of time needed for compilation.
Why is Java Architectural Neutral?
Its compiler generates an architecture-neutral object file format, which makes the compiled code to be executable on many processors, with the presence of Java runtime system
Mention some Java Keywords?
Some Java Keywords are Import, Super, Finally etc.
How do you define a class?
A Java class is a blue print from which individual objects are created. A class can contain fields and methods to describe the behavior of an object.
What is a Local Variable, Instance Variable and Class variable?
Local Variables: Variables defined inside methods, constructors or blocks are called local variables. The variable will be declared and initialized within the method and it will be destroyed when the method has completed.
Instance Variables: Instance variables are variables within a class but outside any method. These variables are instantiated when the class is loaded.
Class Variables: These variables declared with in a class, outside any method, with the static keyword.
Default Byte type value in java?
Default value is 0.
What is a static Variable?
Class variables also known as static variables are declared with the static keyword in a class, but outside a method, constructor or a block.
What is a Classloader?
A Class Loader is a subsystem of JVM that is used to load classes and interfaces. eg.: Bootstrap classloader, Extension classloader, System classloader, plugin classloader etc.
Explain Runtume Polymorphism?
Run time (Dynamic) polymorphism is the polymorphism existed at run-time. Here, Java compiler does not understand which method is called at compilation time. Only JVM decides which method is called at run-time. Method overloading and method overriding using instance methods are the examples for dynamic polymorphism.
Explain static binding and dynamic binding?
The compiler only knows that the type of “a” is Animal ; this happens at compile time, because of which it is called static binding (Method overloading). But if it is dynamic binding then it would call the Monkey class method. Java uses static binding for overloaded methods and dynamic binding for overridden methods.
1) Static binding in Java occurs during Compile time while Dynamic binding occurs during Runtime.
2) private, final and static methods and variables uses static binding and bonded by compiler while virtual methods are bonded during runtime based upon runtime object.
3) Static binding uses Type(Class in Java) information for binding while Dynamic binding uses Object to resolve binding.
3) Overloaded methods are bonded using static binding while overridden methods are bonded using dynamic binding at runtime.
What is a Constructor?
Constructor is a block of code that allows you to create an object of class. This can also be called creating an instance. Constructor looks like a method but it’s not, for example methods can have any return type or no return type (considered as void) but constructors don’t have any return type not even void.
What are different types of constructors?
Default constructor
No-arg constructor
Parameterized Constructor
How to Call a constructor?
To call a constructor use the keyword new, followed by the name of class, followed by parameters if any. For example to create the object of class Mangoe, you can call the constructor like this: new Mangoe()
What are access modifiers?
Access Modifiers are set to access levels for classes, variables, methods and constructors. There are four levels:
Visible package (No modifiers needed here)
Visible to class only (Private to class)
Visible to world (Public)
Visible to the package and all subclasses (Protected)
ParseInt() in Java?
The first argument is interpreted as representing a signed integer in the radix specified by the second argument, exactly as if the arguments were given to the parseInt(java.lang.String, int) method. The result is an Integer object that represents the integer value specified by the string
Can we achieve runtime Polymorphism by data members?
No.
What is Encapsulation and Abstraction?
Encapsulation is hiding the implementation details which may or may not be for generic or specialized behaviors.
Abstraction is the process of generalization: taking a concrete implementation and making it applicable to different, albeit somewhat related, types of data.
Can we use abstract method and final both with a method?
No. Because final methods cannot be overridden.
Can we instantiate abstract class?
Abstract class can never be instantiated.
What is the difference between StringBuffer and StringBuilder?
The difference between StringBuffer and StringBuilder is that StringBuffer is thread-safe. So when the application needs to be run only in a single thread then it is better to use StringBuilder. StringBuilder is more efficient than StringBuffer.
What is a finalize() method?
Finalize() method is defined / called just before an objects final destruction by garbage collector, it is used to make sure that object terminates cleanly.
What is interface in java?
An interface is a special form of an abstract class which does not implement any methods. In Java, you create an interface like this:
interface Interface
{
void interfaceMethod();
}
Can we declare an interface method as static?
Interface methods is abstract by default and static and abstract keywords cannot be used together. Hence No.
Difference between abstract class and interface class?
Abstract | Interface |
Partial implementation | We don’t know anything about implementation, just requirements |
It has an abstract method. An addition, it uses concrete | Every method is public and abstract by default |
No restriction for abstract class method modifiers | It is a 100% pure abstract class |
No restriction for abstract class variable modifiers | If we declare public we cannot declare private and protected |
No restriction to initialize variables | Every interface has public, static and final |
A constructor is not allowed | Instance and static block not allowed |
No restriction to initialize variables | Every variable is static |
Is it possible to define Private and Protected modifiers variables in interfaces?
They both are implicitly public, hence no.
What is a finally block?
A Block that is finally is always executed.